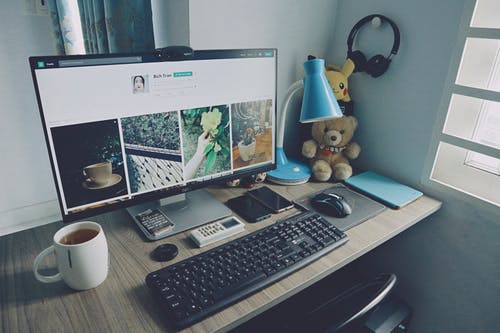
Introduction to Displaying Posts in a Loop in WordPress
Before we dive into how to display any number of posts in a loop, let’s first understand what a loop is. In WordPress, a loop is a PHP code used to display posts on your website. By default, WordPress displays the latest 10 posts in a loop.
A loop consists of two main parts: the query and the loop itself. The query is used to retrieve the posts from the WordPress database, while the loop is used to display these posts on the website.
Why Limit the Number of Posts Displayed in a Loop?
Limiting the number of posts displayed in a loop can improve your website’s performance. Displaying a large number of posts on a single page can slow down your website’s loading speed, which can negatively affect your user experience.
Additionally, displaying too many posts can make your website look cluttered and overwhelming, making it difficult for your visitors to navigate your website.
How to Display a Specific Number of Posts in a WordPress Loop
To display a specific number of posts in a WordPress loop, you can use the posts_per_page
parameter. This parameter allows you to set the number of posts you want to display in a loop.
Here’s an example of how to display five posts in a loop:
<?php
$args = array(
'posts_per_page' => 5
);
$loop = new WP_Query( $args );
if ( $loop->have_posts() ) {
while ( $loop->have_posts() ) {
$loop->the_post();
// Display post content here
}
}
In the above code, we have set the posts_per_page
parameter to 5, which means that only five posts will be displayed in the loop.
Using the pregetposts Function to Display Posts in a Loop
The pre_get_posts
function is used to modify the main WordPress query before it is executed. This means that you can use this function to modify the number of posts displayed in a loop.
Here’s an example of how to use the pre_get_posts
function to display 10 posts in a loop:
<?php
function custom_query( $query ) {
if ( $query->is_home() && $query->is_main_query() ) {
$query->set( 'posts_per_page', 10 );
}
}
add_action( 'pre_get_posts', 'custom_query' );
In the above code, the custom_query
function is used to modify the main WordPress query on the homepage. We have set the posts_per_page
parameter to 10, which means that 10 posts will be displayed in the loop.
Displaying Posts by Category or Tag in a WordPress Loop
You can also display posts by category or tag in a WordPress loop using the cat
or tag
parameter.
Here’s an example of how to display posts from a specific category in a loop:
<?php
$args = array(
'cat' => 5
);
$loop = new WP_Query( $args );
if ( $loop->have_posts() ) {
while ( $loop->have_posts() ) {
$loop->the_post();
// Display post content here
}
}
In the above code, we have set the cat
parameter to 5, which means that only posts from category 5 will be displayed in the loop.
Adding Pagination to Your WordPress Loop
If you have a large number of posts on your website, you can add pagination to your WordPress loop to improve your website’s performance and user experience.
Here’s an example of how to add pagination to your WordPress loop:
<?php
$paged = ( get_query_var( 'paged' ) ) ? get_query_var( 'paged' ) : 1;
$args = array(
'posts_per_page' => 5,
'paged' => $paged
);
$loop = new WP_Query( $args );
if ( $loop->have_posts() ) {
while ( $loop->have_posts() ) {
$loop->the_post();
// Display post content here
}
}
echo paginate_links( array(
'total' => $loop->max_num_pages
) );
In the above code, we have added the paged
parameter to the query to display the correct set of posts on each page. We have also used the paginate_links
function to display pagination links at the bottom of the loop.
Plugins to Simplify Displaying Posts in a WordPress Loop
There are many plugins available in the WordPress repository that can simplify displaying posts in a WordPress loop. Here are some popular plugins:
- WP Show Posts: A powerful plugin that allows you to display posts in a loop with various customization options.
- Post Grid: A lightweight plugin that allows you to display posts in a grid layout.
- Advanced Post List: A plugin that allows you to display posts in a list format with various customization options.
Common Issues and How to Troubleshoot Them
Here are some common issues you may encounter when displaying posts in a WordPress loop and how to troubleshoot them:
- Posts are not displaying in the loop: Make sure that the query is correct and that the loop is set up properly. Check for any syntax errors in your code.
- Posts are displaying in the wrong order: Make sure that you have set the
orderby
andorder
parameters correctly in the query. - Pagination links are not working: Make sure that you have set the
paged
parameter correctly in the query and that you have used thepaginate_links
function to display the pagination links.
Conclusion and Next Steps for Simplifying Your WordPress Site
In this guide, we have learned how to display any number of posts in a WordPress loop. We have also learned how to display posts by category or tag, add pagination to a loop, and troubleshoot common issues.
By following these steps, you can simplify your WordPress site and improve your user experience. If you want to take your WordPress site to the next level, consider using a plugin to simplify displaying posts in a loop.